
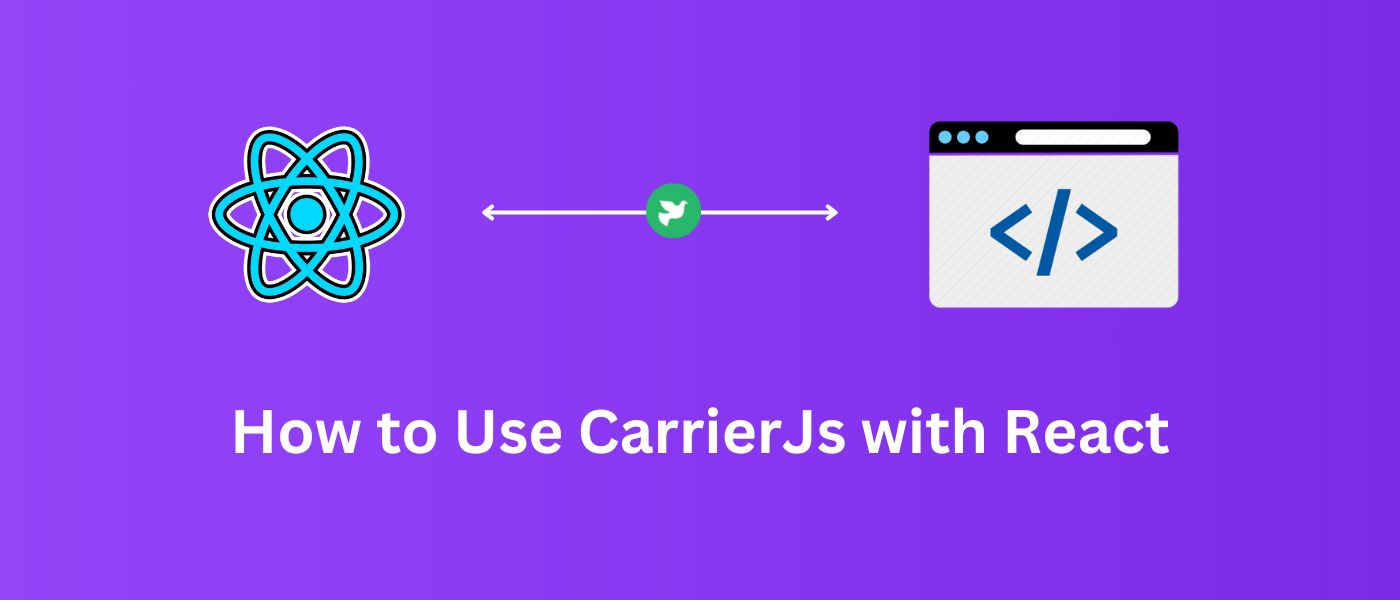
In this blog, I will show you how to use carrierjs javascript library with react framework in very simple steps.
Introduction
Most web applications require fetching data from APIs at some stage in their development. And most of the time web applications/clients send multiple requests to the same API, which increases the load on the server. To reduce the server load we need to implement some caching in web applications.
What are Carriers?
There are many ways of making API calls and caching them in React applications. You can choose either the built-in fetch API and implement a custom cache or third-party libraries. In the latter case, Carrierjs is one of the easiest-to-use HTTP clients available on npm which provides an inbuilt persistent caching feature.
CarrierJs is an open-source, promise-based HTTP client. It uses javascript’s promises to send HTTP requests and manage their responses. It uses the browser’s IndexedDB for persistent caching.
Let’s get started!!
1. Create a React Project
In case you don’t have any existing react projects, create one using the below command:
# Create react project
npx create-react-app carrierjs-react-app # Navigate and start the project server
cd carrierjs-react-app npm start
2. Install Carrierjs
You can install carrierjs using npm in your project –
npm install carrierjs
When the installation has been completed, carrierjs
will be added to your project’s dependencies and you can now import the package into your project.
Open the app.js
the file of your React application (or whichever file you want to use) and import the carrierjs
library at the top:
import carrier from 'carrierjs';
Now it’s ready for you to use in your code.
In the next sections, we’ll walk through how to make some requests.
3. Make a GET Request with caching feature
First, let’s make a GET request using the carrierjs package we just installed.
We are using a fake & free JSON Placeholder service, which provides a fake REST API that returns data for testing.
Add this code to the app.js
or another React file of your choice:
import carrier from 'carrierjs';
import { useEffect } from 'react'; function App() { let fetchData = async () => { try { const res = await carrier.get('https://jsonplaceholder.typicode.com/todos/'); console.log(res); } catch (error) { console.log(error); } } useEffect(() => { fetchData(); }, []) return ( <div> <span>Page Content Goes Here</span> </div> )
} export default App;
After running first time on the browser you will see an API request in the network section and its response in the console section
But if you refresh your browser, now you will see nothing in your browser but this time carrier takes the response from its cache and print the response in the console section.
4. Make GET Request without caching
You only need to pass an extra argument in the carrier.get(URL, true)
method.
Follow below code snippet –
let fetchData = async () => { try { const res = await carrier.get('https://jsonplaceholder.typicode.com/todos/', true); console.log(res); } catch (error) { console.log(error); } }
Now if you refresh your browser, you will always see API requests in your network section. It means carriers are fetching fresh data from the server.
For reference, here’s what the full code for your file should look like when you’re done:
//this code is for without caching request import carrier from 'carrierjs';
import { useEffect } from 'react'; function App() { let fetchData = async () => { try { const res = await carrier.get('https://jsonplaceholder.typicode.com/todos/', true); console.log(res); } catch (error) { console.log(error); } } useEffect(() => { fetchData(); }) return ( <div> <span>Page Content Goes Here</span> </div> )
} export default App;
Feel free to play around with the code and experiment!
Here is the Official Documentation of CarrierJs
Conclusion
We hope this article was helpful in your coding endeavors.
As always, thanks for reading, and happy learning !
L O A D I N G
. . . comments & more!
How to Implement Caching in React Using CarrierJS
Source: Trends Pinoy
0 Comments